Using ESLint as an ES2015 Learning Tool
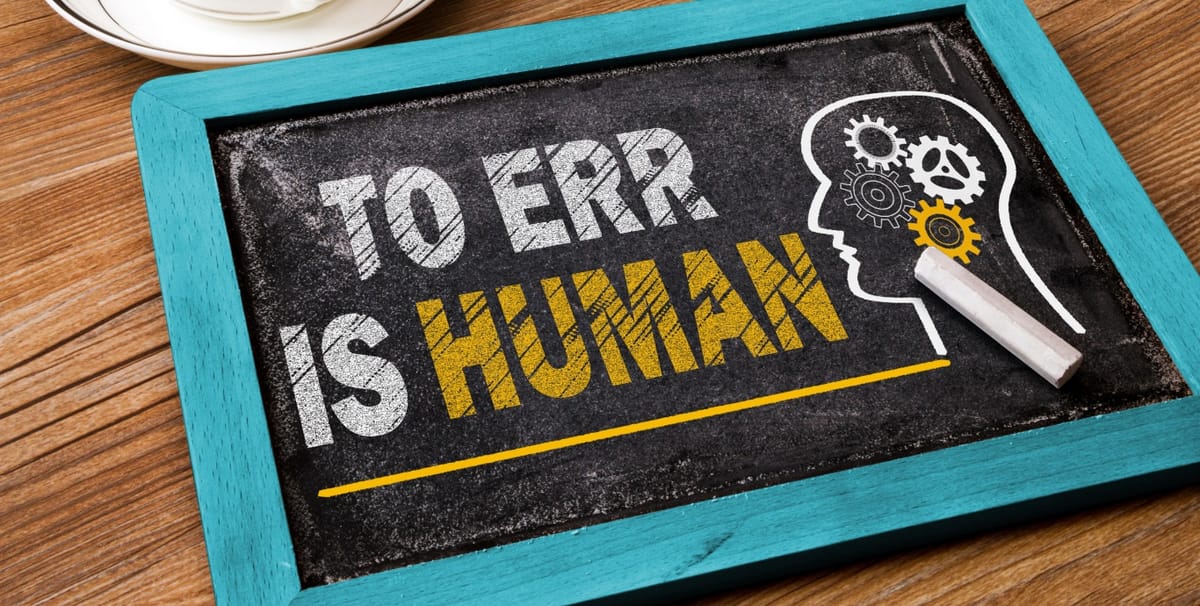
I have used various linting tools to keep code consistent across teams. A few weeks back, I started using ESLint with my website and hobby projects. Most of the projects used plain old ES3 syntax. I expected some nagging about semicolon and curly brace placement, but, to my surprise, ESLint provided many more usage suggestions than stylistic changes to the ECMAScript. In this blog post, I'll go through ESLint's suggestions and how the result was better than the original code.
Benefits of linting
"Lint" may also refer to general syntactic discrepancies, especially in interpreted languages like JavaScript and Python. For example, modern lint checkers are often used to find code that doesn't correspond to certain style guidelines. They can also provide simple debugging for common or hard-to-find errors such as heisenbugs. -Wikipedia
For a competent ES2015 developer who already knows how to use new language features, ESLint brings joy. The reason is that writing greenfield ES2015 source code is easier than spotting things to improve in a legacy application. ESLint can not only help you to identify things to improve, but can also fix some of the issues for you, saving you a few keystrokes! The list of ES2015 issues that ESLint can automatically repair (ten at the time of writing) is not long, but, combined with stylistic auto-fixes, ESLint can save time.
Quick introduction to ESLint
ESLint is an open source project created by Nicholas C. Zakas in June 2013. Its goal is to provide a pluggable linting utility for JavaScript. -eslint.org
Instructions for installing ESLint are here. "Rule" is the ESLint term for a single check. Each rule has a string identifier (for example, "no-fallthrough") and a configuration for that rule. The documentation of each rule is where ESLint shines, see no-fallthrough, for example. Rules and their configurations are located in the file .eslintrc.
The list of possible rules is long, and starting from scratch may be overwhelming. ESLint configuration supports extending an existing configuration. After this extension, you can override the extended settings according to your needs.
Getting started
After I checked the Airbnb JavaScript style guide and found that I agreed with most of it, choosing the Airbnb Base ESLint configuration as my starting point was easy.
With a single npm command you can install all the required packages:
npm install --save-dev eslint-config-airbnb-base eslint-plugin-import eslint
To use the Airbnb configuration as our base, we need to modify .eslintrc:
{
"extends": "airbnb"
}
Putting ESLint to work
There are several ways to use ESLint on a daily basis:
- add ESLint to your development process as a build step (Gulp, Grunt, npm script, Broccoli, etc.)
- let your text editor run ESLint
- run ESLint manually from the command line
I'll demonstrate ESLint ES2015 features using the Sublime Text editor and the SublimeLinter plugin. For a production grade project with multiple developers, there would be discussion (possibly long) about which rules to use and what should happen when an ESLint build task spots something.
I opened a simple JavaScript utility file that I use on my website.

Expected method shorthand was the first ESLint error.
module.exports = {
removeClass: function (el, className) {
..
}
}
This error can be fixed automatically:
module.exports = {
removeClass(el, className) {
..
}
}
Ten characters less to type for each function in an object literal. Win!
The following refactoring changes an anonymous method so that it uses arrow syntax.

Note that the anonymous method's behavior isn't similar to arrow functions behavior. Arrow syntax doesn't bind its own this
; it captures the enclosing context's this
value instead. The source code I used had only a console.log
statement, and the anonymous function didn't refer to this
, so the code could be refactored safely.
If you want to learn about the var, let, and const keywords in a practical way, you can use ESLint to help you identify proper usage.

let allows you to declare variables that are limited in scope to the block, statement, or expression in which they are used. This is different from the var keyword, which defines global variables or variables that are local to an entire function regardless of block scope. -MDN
In the following example, ESLint noticed that var could be changed to let. After I changed var to let, ESLint explained that the keyword could be const because no one would be changing the value. This is perfectly valid refactoring; if the variable will not be reassigned, then const, the strictest definition, is a perfect choice.
Conclusion
I've shown a few practical examples of how ESLint can help to refactor an existing ES3 codebase. ESLint is a valuable learning tool when you know the existing codebase and can see the improvements in the resulting code.
The ESLint website has excellent documentation on each rule and the basics of ESLint. If you have noticed a new rule that could be applied in your organization and want to dig deeper, you can write your own rule or plugin.
I would like to hear your experiences with linting and code quality!