Riot custom tag by example
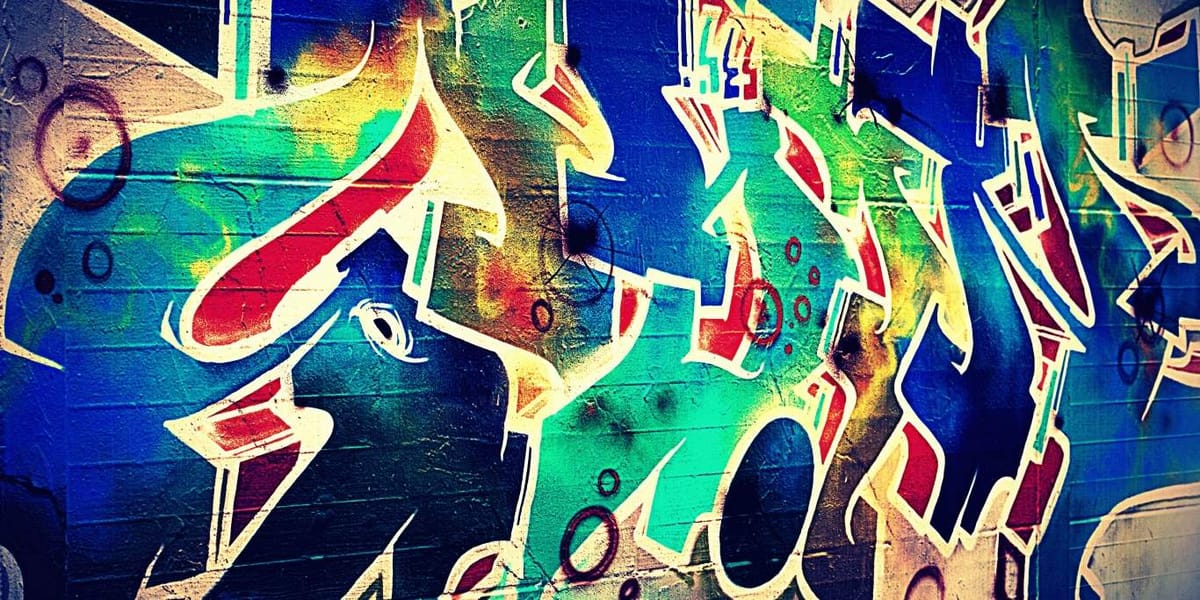
Riot
Riot brings custom tags to the browsers (even on IE8). This means that we can write reusable components that contain all the required structure (HTML), logic (JavaScript) and (optionally) styling (CSS) inorder to use the component.
These custom tags doesn't need any special prefixes. Plain and simple <fancypizzapicker></fancypizzapicker>
is valid.
From the FAQ section of Riot-website:
Riot is against the current trend of boilerplate and unneeded complexity. We think that a small, powerful API and concise syntax are extremely important things on a client-side library.
This warms my heart.
Creating a custom tag
To actually implement new tag is pretty straightforward. As an example I forked "Flat Clock"
by Joseph Shambrook and converted it to a custom tag.
First we need a new file and there we need to have the custom tag.
<clock>
</clock>
Naming the file:
One convention is to use filename extension tag to identify custom tag. We started using Riotify Gulp-plugin and it requires files to have tag extension, so that's how convention was created for us. :)
Another thing:
Don't put any class definitions on the custom element itself only on it's content. At least when you're compiling the tag (more about compiling in the next post) you'll get parser error Unexpected token (2:0) if you accidently put a class name.
When we have created the file and added custom tag then we need to start defining the HTML content for it.
<clock>
<div class="clock">
<div class="hour"></div>
<div class="minute"></div>
</div>
</clock>
Add some logic
<clock>
<div class="clock">
<div class="hour"></div>
<div class="minute"></div>
</div>
</clock>
function clock() {
var t = moment(),
a = t.minutes() * 6,
o = t.hours() % 12 / 12 * 360 + (a / 12);
$(".hour").css("transform", "rotate(" + o + "deg)");
$(".minute").css("transform", "rotate(" + a + "deg)");
}
function refreshClock() {
clock(), setTimeout(refreshClock, 1000)
}
refreshClock();
To use the newly created custom tag you need to have a link the file and also to the Riot library.
<script src="clock.tag" type="riot/tag"></script>
<script src="https://cdn.jsdelivr.net/g/riot@2.0.12(riot.min.js+compiler.min.js)"></script>
Then we are ready initialize the component.
riot.mount('clock')
See full example below. The CodePen example has the custom tag definition inside <script type="riot/tag">
instead of new file.
See the Pen Flat Clock by Tatu Tamminen (@ttamminen) on CodePen.
Embedded JavaScript
Linking your custom tags separately might be okay for one or two custom tags, but after that there starts to be too many requests. Next blog post will explain how to use Riot's Compiler (in a Browserify + Riotify + Gulp setup) to deliver your custom tags efficiently.