How to use Compose.io API to dynamically create databases using NodeJS
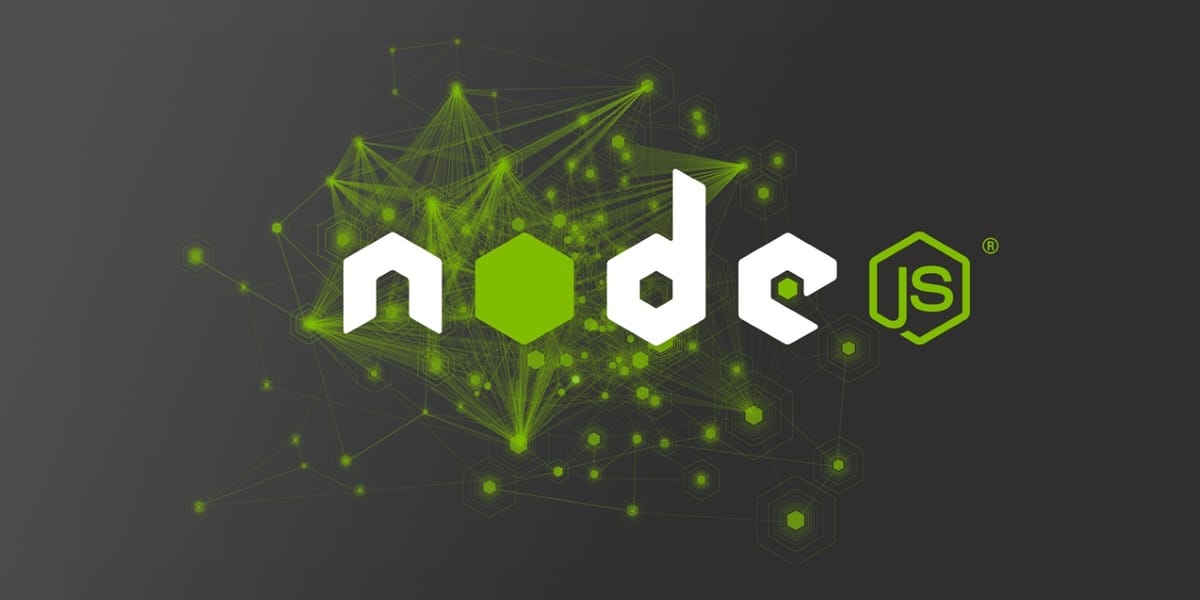
The project I'm working on has a database per client architecture. The database is created when a client registers to the service. In the first phase, we did this manually, but switching to the automatic process was just a matter of time.
In this article, we're going to use Compose.io (ex-MongoHQ) service and it's API to create a database instance. To simplify things we're using Personal Access Tokens as an authorization method instead of OAuth.
Create token
The first step is to create Personal Access Token. This can be done easily in the Compose.io control panel.
After giving a name for the token, you'll get generated token that you should store for later use.
Let's play with the API..
Get accounts
Now that we have a token we can step by step go further into the data. Let's try to get a list of accounts we have access.
I am using Google Chrome extension called DHC to test the API before writing actual code.
Note: you need to provide Accept-Version
otherwise you'll get 404
If everything went smoothly you'll get 200 OK.
Get deployment and list of databases
Now that we have account information we can test that we can get single deployment from an account. The account information is in the url part just before deployment name (or id).
Let's try fetching a list of databases.
If everything went smoothly you should see a list of your databases + few internal ones:
We can later use this API call to verify that database has been created.
Create database
I had to search a bit to get this done. There isn't "database create" API call anymore, instead, what you need to do is create a new collection and give a database name which will be automatically created.
After successful POST, you should see a new database in the Compose.io dashboard.
Show me the code!
I have created a working example that creates a database to Compose.io service. It uses two libraries: request and Bluebird to make a code bit more readable.
The interesting part is this block of code:
function createCollection(conf, name) {
var baseUrl = 'https://api.compose.io/';
var createCollectionUrl = 'deployments/' +
conf.accountName + '/' +
conf.deploymentName +
'/mongodb/' +
conf.databaseName +
'/collections';
return request.postAsync({
baseUrl: baseUrl,
url: createCollectionUrl,
headers: {
'Authorization': 'Bearer ' + conf.token,
'Accept-Version': conf.apiVersion
},
json: true,
body: {
name: name
}
});
Note: I was a bit lazy and used string concatenation instead of ES6 string interpolation
The example shows how to provide a necessary header, url and body data for the POST.
Adding other requests is easy after one working request.
Check out the repository and let me know if you had success or failure with it!