Better React linting by switching from JSHint to ESLint
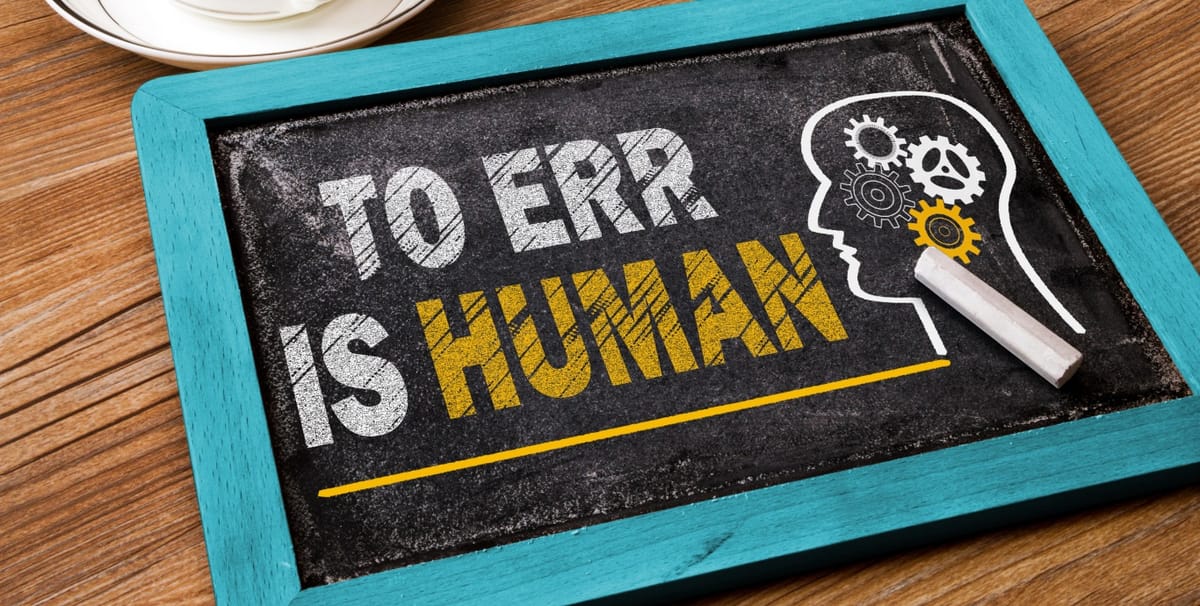
JSHint - the JavaScript Code Quality Tool, as they call it, has served me well for past year. I enjoyed Sublime integration via SublimeLinter plugin, and the plugin had the right balance of rules: not too strict when I knew what I was doing and not too loose to miss a possible error. One day I hit an unpassable wall. I started to learn React.
React + JSX + JSHint = Problems
While learning React, I began to use ES2015 language features such as import, const, and class. JSHint can tackle those easily using configuration option "esnext": true
. The configuration option might change in the future releases as the blog post explains.
JSHint 3 will not expose an esnext option; it will instead support esversion: 6.
Makes sense, next is a moving target.
Unfortunately, JSX was not supported at all, and I didn't find a clean solution to fix the situation. False errors will ruin the whole experience, so it was time to look alternatives.
React + JSX playing nice with ESLint
It's a promising start for searching alternatives as ESLint tagline says: "The pluggable linting utility for JavaScript and JSX". Installation was a straight forward npm install -g eslint
. Just remember to run as an administrator if you're doing the global installation.
In the project folder, I ran an init command eslint --init
which asks few questions and finally creates a configuration dot-file. The questions " Do you use JSX?", "Are you using ECMAScript 6 features?" and "Do you use React?" are the ones we're now interested in, as the blog post covers those use cases.
It's good to test that ESLint is working by giving it an input file and check the result.
ESLint configuration
Initialization creates a configuration file that is very similar to the JSHint equivalent.
{
"rules": {
"indent": [
2,
4
],
"quotes": [
2,
"single"
],
"linebreak-style": [
2,
"windows"
],
"semi": [
2,
"always"
]
},
"env": {
"es6": true,
"browser": true
},
"extends": "eslint:recommended",
"ecmaFeatures": {
"jsx": true,
"experimentalObjectRestSpread": true
},
"plugins": [
"react"
]
}
React support is done using a plugin. If you did global ESLint install, then the plugin should also be globally installed npm install -g eslint-plugin-react
.
The plugin contains plenty of React specific linting rules, such as prefer-es6-class, no-unknown-property etc.
Interestingly jsx: true
is in the ecmaFeatures
.
After installing ESLint and ESLint-plugin-react, I still got an error: Parsing error: Illegal import declaration
I was missing "modules": true
setting inside ecmaFeatures
.
Running ESLint
The main thing is that the command line tool eslint
doesn't have any problems before installing any editor plugins as they all rely on it. I am currently using Sublime Text, and it's plugin SublimeLinter-eslint. The installation is straightforward once you have ESLint installed.
Which is same as command line output:
Next steps
Working linting setup is a base for productive JavaScript development. The installation on a local machine developer can do under 15 minutes, so there isn't any excuse to do it. When you're working with a team, you need to agree on the linting rules and share the config file using preferred source control system.
Add it also part of the build process to make sure its run even if editor plugin doesn't work or manual start of JSLint is forgotten by developers.