Adding compiled Riot tags to your Gulp + Browserify build
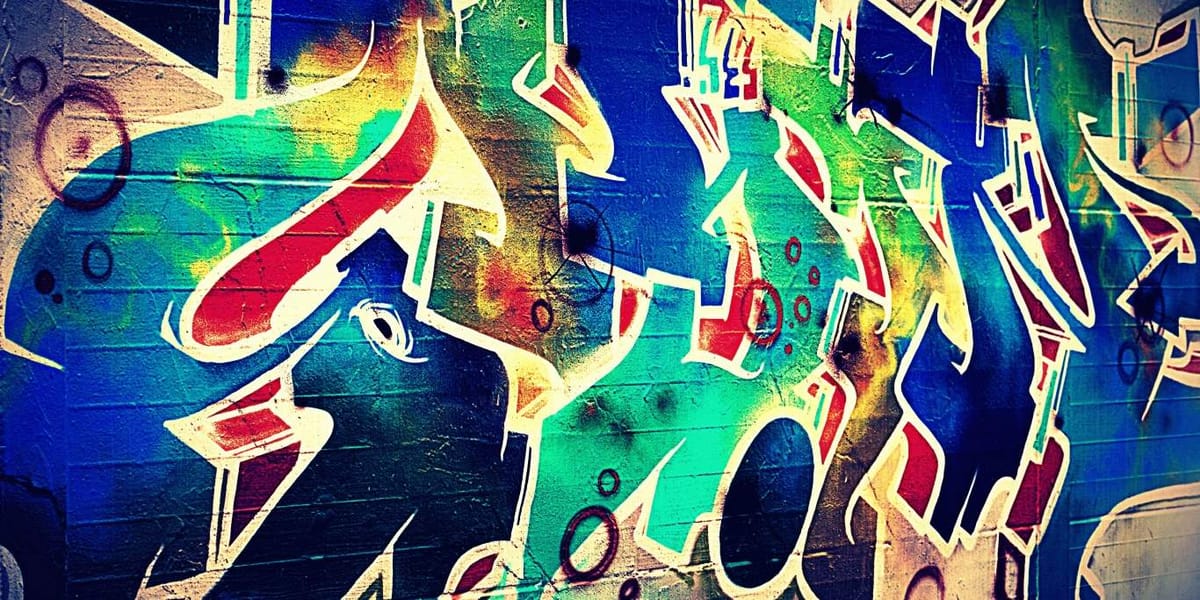
What are we trying to achieve?
In the previous blog post (titled: Riot compiler explained) we briefly touched the topic of adding Riot tag compilation to the build process. This blog post will explain that aspect in more detail.
In this blog post we're going to use Gulp and Browserify to build JavaScript asset to the client that includes compiled Riot tags. The end goal is to have single JavaScript file that includes your own source + all it's dependencies. It will be assumed that Node and npm properly installed.
Instead of Gulp you could use Grunt or Broccoli and instead of Browserify there is an alternative called webpack.
The complete example can be found from GitHub.
Getting started
First thing is to install required npm packages:
npm install --save-dev gulp riot riotify browserify vinyl-source-stream
Create a file called Gulpfile.js and put following content in it.
var gulp = require('gulp');
gulp.task('default', function() {
});
Create a directory for your scripts and one for tags. After that you should have following file structure.
scripts/
tags/
Gulpfile.js
package.json
At this point running command gulp
shouldn't give you any errors.
Browserify Require's
We should have a Browserify entry file that requires Riot and single tag. I created a JavaScript file named main.js to the scripts folder:
var riot = require('riot');
require('../tags/clock.tag');
riot.mount('clock');
First we require
Riot library then we require
the compiled tag and finally we "replace" any occurences of <clock>
with the actual implementation of a custom tag.
Place your custom tag to the tags directory or get an example from here.
Gulpfile
Our almost empty Gulpfile requires some more fat on top of bones.
var gulp = require('gulp');
var browserify = require('browserify');
var riotify = require('riotify');
var source = require('vinyl-source-stream');
gulp.task('browserify', function () {
return browserify({
debug: true,
// this is an array of all entry points
// where Browserify starts to look for
// dependencies
entries: ['./scripts/main.js'],
// list of transforms that are supported
// riotify needs .tag file name extension
// to support tag compilation
transform: [riotify]
}).bundle()
// take the end result and place it to dist folder
.pipe(source('main.bundle.js'))
.pipe(gulp.dest('./dist/'));
});
gulp.task('default', ['browserify']);
When you run this task using Gulp you should see something like this:
PS C:\GH\riot-tag-build> gulp
[14:45:39] Using gulpfile C:\GH\riot-tag-build\Gulpfile.js
[14:45:39] Starting 'browserify'...
[14:45:39] Finished 'browserify' after 153 ms
[14:45:39] Starting 'default'...
[14:45:39] Finished 'default' after 15 μs
Checking the results
The interesting thing is in the dist
directory. You should see a file main.bundle.js
. It should contain Riot.js (from the npm module), main.js and the compiled version of the tag. All the required files/libraries in one file.
In my case the tag was in the bottom of the bundle file and it looks familiar if you read the Riot compiler explained.
Next steps would be adding JavaScript minification, JSHint/JSLint tasks etc. to the process according to your needs.